Add / remove user to SharePoint groups with PowerShell
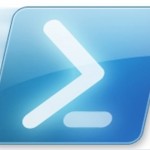
In SharePoint site you can see usually a lot of groups. Sometimes administrator gets a task to add user to several groups. If it’s 3-5 groups you can do it manually, but if you need to add user to 30 groups? I’m too lazy to do it manually and too smart not do it at all.
PowerShell Script will help us. But because this script is more than one string I’ll use PowerShell ISE (read about how to add it in How to add PowerShell ISE from PowerShell).
So let’s start out PowerShell ISE and connect SharePoint CMD-let:
if ((Get-PSSnapin "Microsoft.SharePoint.PowerShell" -ErrorAction SilentlyContinue) -eq $null) { Write-Host "Connect Sharepoint cmd-Let" Add-PSSnapin Microsoft.SharePoint.PowerShell }
Let’s enter site collection url, username for inserting to groups and get array of destination SharePoint groups. For example, let this groups contain a string "admin"
$url = "http://alexey-pc7" $userName = "Alexey-PC7\testuser1" $site = new-object Microsoft.SharePoint.SPSite($url) $web = $site.OpenWeb() $groups = $web.sitegroups write-host "------get array of groups which contain a string "admin"-----" $myGroups = @(); foreach($group in $groups) { if($group -match "admin") { $myGroups += $group } }
Go through each element of array and add user to SharePoint group
foreach ($gr in $myGroups) { #add user to SP Group Set-SPUser -Identity $userName -web $url -Group $gr write-host "User " $userName "added to " $gr }
And if you made a false and, for example, entered wrong username, you can easily remove user from SharePoint groups with this PS-code:
foreach ($gr in $myGroups) { $theGroup = $web.SiteGroups[$gr] $theUser = $web.AllUsers.Item($userName) #Remove user from SP Group $theGroup.RemoveUser($theUser); write-host "User " $userName " removed from " $gr }
If you don’t want to collect these pieces here’s a full version:
if ((Get-PSSnapin "Microsoft.SharePoint.PowerShell" -ErrorAction SilentlyContinue) -eq $null) { Write-Host "Connect Sharepoint cmd-Let" Add-PSSnapin Microsoft.SharePoint.PowerShell } $url = "http://alexey-pc7" $site = new-object Microsoft.SharePoint.SPSite($url) $web = $site.OpenWeb() $groups = $web.sitegroups $userName = "Alexey-PC7\testuser1" write-host "--------------" $i = 0; $myGroups = @(); foreach($group in $groups) { if($group -match "admin") { $myGroups += $group } } foreach ($gr in $myGroups) { write-host $gr #add user to SP Group Set-SPUser -Identity $userName -web $url -Group $gr $theGroup = $web.SiteGroups[$gr] $theUser = $web.AllUsers.Item($userName) #Remove user from SP Group # $theGroup.RemoveUser($theUser); write-host "User " $userName "added to " $gr }
If there are non-english letters in a group name or if the group you need is on another site (but in this site collection), then you can have error on Set-SPUser command. In this case I can advice you to use another command $web.SiteGroups[group name].AddUser(user)
foreach ($gr in $myGroups) { Write-Output "Группа: $gr " #alternative way to add user to SP Group $theUser = $web.AllUsers.Item($userName) $web.SiteGroups[$gr].AddUser($theUser) Write-Output "User $userName added to $gr" }
To cater for users who are not already associated with the site collection I added the following before the for loop that calls Set-SPUser
# user added to the site collection first, will error if user isn’t already associated with the site collection
$web | New-SPUser –UserAlias $userName
Comment by chris johnston — November 5, 2013 @ 3:05 pm
Awesome!!! Thanks
Comment by prateek rawat — July 23, 2015 @ 9:58 am