Add hyperlink to GridView programmatically
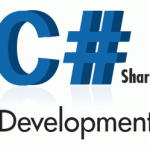
GridView is a powerful and easy in use tool in Asp.net, especially if you know how to use it. There are a lot of tutorials how to add dynamic hyperlink column to GridView not programmatically, but this tutorial is about how to add hyperlink to GridView programmatically (in code-behind).
Initial data:
You have a class CEvent and collection List<CEvent>:
public class CEvent { public int eventId { get; set; } public string title { get; set; } public string reference { get; set; } public string company { get; set; } }
And you want to have a column in your GridView with a hyperlink of this format:
<a href="corpevent.aspx?evid=103&company=MyComp">MyComp</a>
Solution:
In a lot of tutorials it’s written how to add hyperlink column in GridView control via designer in .aspx page. They look somehow like this:
<asp:GridView ID="GridView1" runat="server"> <Columns> <asp:BoundField DataField="eventId" /> <asp:HyperLinkField DataNavigateUrlFields="eventId, company" DataNavigateUrlFormatString="WebForm1.aspx?eventId={0}&company={1}" /> </Columns> </asp:GridView>
It’s a good way. But there are a lot of cases, when you have to write GridView properties and add columns from code-behind. It wasn’t very easy for me at first.
I’ve decided to describe HyperLinkField equivalent to .aspx syntax:
gv.Columns.Add(new HyperLinkField() { DataNavigateUrlFields = "eventId, company", DataNavigateUrlFormatString = "corpevents.aspx?evid={0}&company={1}", HeaderText = "Open" });
But I’ve got this error:
Error 2 Cannot implicitly convert type 'string' to 'string[]'
The reason is that value for "DataNavigateUrlFields" must be a string array, that’s why I had to change some code. One of correct ways to add hyperlink to GridView programmatically is below:
string[] dataNavigateUrlStrings = { "eventId", "company" }; gv.Columns.Add(new HyperLinkField() { DataNavigateUrlFields = dataNavigateUrlStrings, DataNavigateUrlFormatString = "corpevents.aspx?evid={0}&company={1}", Text = "Open", HeaderText = "Link" });