C#. Best practice for OleDBConnection
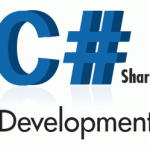
It’s a little example, or may be template, of correct code for work with MSSQL server using OleDbConnection. There are two parts you should remember about - connection string and OleDB commands. The most difficult part of this is the connection string. It took me a lot of my time until I studied how to write this string easily.
I have a "secret" knowledge how easily write the text of connection string and even to try it. This post is for registered users only (https://markimarta.com/c/how-easily-write-the-text-of-connection-string/).
Below is the part of C# code to work with OleDbCommand:
static string connSQL = "Provider=SQLOLEDB.1;Password=Pass@word1;Persist Security Info=False;User ID=searchUser;Initial Catalog=ArchiveDB;Data Source=sqlserver\\DBName"; string sqlCommand = String.Format(@"SELECT ID, message FROM mytable WHERE ID = '{0}' ORDER by ID desc", "123"); OleDbConnection oledbCnn = new OleDbConnection(connSQL); string tableContent = ""; try { oledbCnn.Open(); OleDbCommand cmd = new OleDbCommand(sqlCommand, oledbCnn); cmd.CommandTimeout = 150; OleDbDataReader reader = cmd.ExecuteReader(); while (reader.Read()) { reader.GetValue(1); } reader.Close(); } catch(Exception ex) { } finally { oledbCnn.Close(); }