Get all IP addresses from text C#
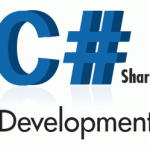
Using "System.Text.RegularExpressions" in C# you have a lot of abilities to work with text. One of practice of using System.Text.RegularExpressions is working with IP addresses.
For examples I’ll use this string:
network-plan: 0.0.4.0 255.255.255.0 PART 256 16/60/240 8 PRI dial up modems network-plan: 0.0.5.0 255.255.255.0 PART 256 0/60/240 8 PRI dial up modems network-plan: 0.0.6.0 255.255.255.192 YES 64 10/16/35 LAN -mail and DNS servers network-plan: 0.0.6.64 255.255.255.192 YES 64 15/25/40 LAN -NOC & Ops management network-plan: 0.0.6.128 255.255.255.240 YES 16 5/11/11 LAN -Name based web hosting network-plan: 0.0.6.144 255.255.255.240 YES 16 0/8/8 LAN -DNS servers network-plan: 0.0.6.160 255.255.255.240 YES 16 4/6/12 loopback router interfaces
Start data:
string strWithIp = @"network-plan: 0.0.4.0 255.255.255.0 PART 256 16/60/240 8 PRI dial up modems network-plan: 0.0.5.0 255.255.255.0 PART 256 0/60/240 8 PRI dial up modems network-plan: 0.0.6.0 255.255.255.192 YES 64 10/16/35 LAN -mail and DNS servers network-plan: 0.0.6.64 255.255.255.192 YES 64 15/25/40 LAN -NOC & Ops management network-plan: 0.0.6.128 255.255.255.240 YES 16 5/11/11 LAN -Name based web hosting network-plan: 0.0.6.144 255.255.255.240 YES 16 0/8/8 LAN -DNS servers network-plan: 0.0.6.160 255.255.255.240 YES 16 4/6/12 loopback router interfaces"; string pattern = @"(\d\d?\d?\.\d\d?\d?\.\d\d?\d?\.\d\d?\d?)"; Regex re = new Regex(pattern, RegexOptions.IgnoreCase);
Get the first IP address:
Match firstMatch = re.Match(strWithIp); Console.WriteLine("The First IP address is: " + firstMatch.ToString());
Get the first IP address after 50 symbol:
Match offsetMatch = re.Match(strWithIp, 50); Console.WriteLine("First IP address after 50 symbol is: " + offsetMatch.ToString());
Get all IP addresses:
Masks are here too because they also match our Regular expression.
MatchCollection mc = re.Matches(strWithIp); foreach (Match mt in mc) { Console.WriteLine(mt); }