Get images from tag 'img src' from string variable
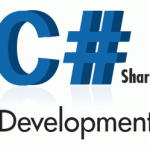
Here's a useful snippet in C#. This method gets all the images from variable type String. It can be HTML or XML text with tags <img src="path_to_image">
public static List<Uri> FetchImagesFromString(string htmlSource) { List<Uri> links = new List<Uri>(); string regexImgSrc = @"<img[^>]*?src\s*=\s*[""']?([^'"" >]+?)[ '""][^>]*?>"; MatchCollection matchesImgSrc = Regex.Matches(htmlSource, regexImgSrc, RegexOptions.IgnoreCase | RegexOptions.Singleline); foreach (Match m in matchesImgSrc) { string href = m.Groups[1].Value; links.Add(new Uri(href)); } return links; }