Multiple file upload in Asp.net
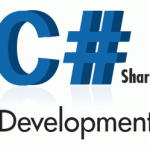
To add multiple upload control and set up handler for it is quite easy in Visual Studio 2013.
Add web.form page to your project, for example "Default.aspx" and add between "<form id="form1" runat="server"> <div>" and "</div></form>" this code:
<h1>Upload Multiple Files</h1> <asp:FileUpload ID="fileUpload1" AllowMultiple="true" runat="server" /> <br /> <asp:Button ID="btn" runat="server" Text="Upload" OnClick="btn_Click" />
In codebehind add this:
using System.IO; ..... protected void btn_Click(object sender, EventArgs e) { HttpFileCollection fileCollection = Request.Files; for (int i = 0; i < fileCollection.Count; i++) { HttpPostedFile uplFile = fileCollection[i]; string fileName = Path.GetFileName(uplFile.FileName); if (uplFile.ContentLength > 0) { uplFile.SaveAs(Server.MapPath("~/Files/") + fileName); } } }