One action on events for several buttons or controls with jQuery
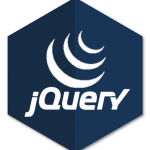
There are 5 buttons on a page. If you click 1, 3 or 4 there must be one action, for 2 and 4 another one. You don’t have to write the same action for each control, like this:
$(document).ready(function () {
$('#button1').on('click', function () {
console.log('good button clicked');
});
$('#button3').on('click', function () {
console.log('good button clicked');
});
$('#button4').on('click', function () {
console.log('good button clicked');
});
$('#button2').on('click', function () {
console.log('wrong button clicked');
});
$('#button5').on('click', function () {
console.log('wrong button clicked');
});
});
Of course, it’s not comfortable and compact record.
You can easily improve the code with 'add()' method.
$('#button1').add('#button3').add('#button4').on('click', function () {
console.log('good button clicked');
});
$('#button2').add('#button5').add('#button5').on('click', function () {
console.log('wrong button clicked');
});
There’s also another way to write the code, but it’s not universal. You can use it only when you know IDs of buttons.
$('#button1, #button3, #button4').on('click', function () {
console.log('good button clicked');
});
With method ‘add()’ you can use variables to get controls.
$ctrl1 = $('#button1');
$ctrl2 = $('#button3');
$ctrl3 = $('#button4');
$ctrl1.add($ctrl2).add($ctrl3).on('click', function () {
console.log('good button clicked');
});