Trick how to make Select control as readonly using jQuery but not disabled
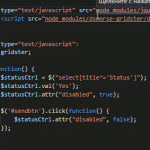
There's a control <select> on your page and you want to use it's value as selected and don't want to allow user to change it. Unfortunately, there's no attribute "readonly" for select as for <input> ones. You can use only attribute "disabled", but in this case its value will not be sent. But there's a trick how to do it.
First you should add attribute "disabled" and set it to "true". In this case after sending your form the value will not be sent to a server. But you can change the value of attribute before sending the form! You should just set the value of attribute "disabled" to false.
For example:
<!-- This is html code of the form --> <select name="tt" title="Status"> <option value="No">No</option> <option value="Yes">Yes</option> <option value="May be">May be</option> </select> <br /> <input type="Submit" id="sendbtn" value="Send"> <!-- This is javascript --> <script type="text/javascript" src="node_modules/jquery/dist/jquery.min.js"></script> <script type="text/javascript"> $(function() { $statusCtrl = $("select[title^='Status']"); $statusCtrl.val('Yes'); $statusCtrl.attr("disabled", true); $('#sendbtn').click(function() { $statusCtrl.attr("disabled", false); }); }); </script>
So, you should change attribute "disabled" value just before submitting.
For example, for SharePoint forms it you should override function OnPreSaveItem().