Remove all the permissions for SharePoint SPWeb, SPList or SPListItem
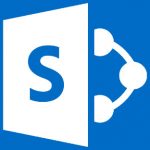
I'm trying to remove all permissions from a list item in SharePoint 2010. There was a lot of permissions because of the "Limited access". I couldn't check all items because of the constraint of maximum selected items. This script is 100% compatible with SharePoint 2013 and SharePoint 2016.
That's why I decided to write a PowerShell script to remove all the permissions from SharePoint object. This can be SPWeb, SPList or SPListItem.
This solution is not the fastest, it simply removes permissions step by step. It can be used if you have less than 100 permissions. In other cases it will be too long. This script can be written by SharePoint junior 🙂
cls if ((Get-PSSnapin "Microsoft.SharePoint.PowerShell" -ErrorAction SilentlyContinue) -eq $null) { Add-PSSnapin Microsoft.SharePoint.PowerShell } function RemoveRoleAssignments($item) { #break inheritance if ($item.HasUniqueRoleAssignments -eq $False) { $item.BreakRoleInheritance($true) } $cpt = $item.RoleAssignments.Count -1 for($i = $cpt; $i -ge 0; $i--) { $item.RoleAssignments.Remove($i) } } $spwebUrl = 'http://spdev/apps/' $spweb = Get-SPWeb $spwebUrl $splist = $spweb.Lists["ServiceApps"] $item = $splist.GetItemById(4) #Remove permissions from SharePoint List Item RemoveRoleAssignments -item $item #Remove permissions from SharePoint List RemoveRoleAssignments -item $splist #Remove permissions from SharePoint SPWeb RemoveRoleAssignments -item $spweb
Here's another version of script. It uses the features of SharePoint. This script will leave only one permission for the item. It cleans all the permissions. Here's another function to remove all the permissions from SharePoint SPWeb, SPList, SPListItem. It checks if SPItem has unique permissions. If it does, then it breaks them all. If no, then it makes the item to inherit all the permissions from the parent and in the next moment it also breaks all the permissions.
cls if ((Get-PSSnapin "Microsoft.SharePoint.PowerShell" -ErrorAction SilentlyContinue) -eq $null) { Add-PSSnapin Microsoft.SharePoint.PowerShell } function RemoveRoleAssignments($item) { #break inheritance if ($item.HasUniqueRoleAssignments -eq $false) { $item.BreakRoleInheritance($false) } else { $item.ResetRoleInheritance() $item.BreakRoleInheritance($false) } } $spwebUrl = 'http://spdev/apps/' $spweb = Get-SPWeb $spwebUrl $splist = $spweb.Lists["ServiceApps"] $item = $splist.GetItemById(3) #Remove permissions from SharePoint List Item RemoveRoleAssignments -item $item #Remove permissions from SharePoint List RemoveRoleAssignments -item $splist #Remove permissions from SharePoint SPWeb RemoveRoleAssignments -item $spweb