Nice way to store Connection strings for external databases in Secure Store SharePoint 2019
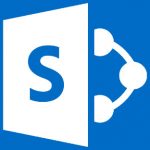
How to store credentials? It’s always a question because nobody wants to demonstrate the passwords, neither for the databases, nor for web services or anything else. SharePoint has a built-in tool to store a sensitive data – technical user accounts, databases or any data like this. Here is how to create variables in the secure store.
It won't take you much time to use Secure Store. It's really easy.
Step 1. Go to Mange Service applications
Step 2. Open Secure Store Service.
Step 3. Press New in Ribbon and fill the following form
Step 4. Enter the properties for you variable and their types.
Step 5. Enter the Administrators and members of the Secure Store Target Application. The first one can manage the Secure Store Target Application, others can use it.
Step 6. Set Credentials to the Secure Store Target Application
Choose "Set Credentials"
Step 7. And fill the form with the values
That's all. If you want to modify the Secure Store Target Application, you can edit it.
So, you have created a Secure Store Target Application and now you want to use it. For the demo, I will write down the methods in C# how to get the values and concatenate the SQL Connection string. You can add them into your class or application. Don't forget to add References to your solution.
using Microsoft.Office.SecureStoreService.Server;
using Microsoft.SharePoint;
using Microsoft.SharePoint.Administration;
using System.Runtime.InteropServices;
using System.Security;
// ....
public static string getSQLFromSecureStore()
{
string SQL = "";
SPAdministrationWebApplication webApp = SPAdministrationWebApplication.Local;
SecureString securePwd = new SecureString();
ISecureStoreProvider provider = SecureStoreProviderFactory .Create();
if (provider == null)
{
throw new InvalidOperationException("Unable to get an ISecureStoreProvider");
}
ISecureStoreServiceContext providerContext = provider as ISecureStoreServiceContext;
providerContext.Context = SPServiceContext.GetContext(webApp.Sites[0]);
using (SecureStoreCredentialCollection creds = provider.GetCredentials("SQLConnectionStringDev"))
{
SQL = GetStringFromSecureString(creds[0].Credential) + "User ID=" + GetStringFromSecureString(creds[1].Credential) + ";Password=" + GetStringFromSecureString(creds[2].Credential);
}
return SQL;
}
private static string GetStringFromSecureString(SecureString secStr)
{
if (secStr != null)
{
IntPtr pPlainText = IntPtr.Zero;
try
{
pPlainText = Marshal.SecureStringToBSTR(secStr);
return Marshal.PtrToStringBSTR(pPlainText);
}
finally
{
if (pPlainText != IntPtr.Zero)
{
Marshal.FreeBSTR(pPlainText);
}
}
}
return null;
}
// ...
public Database(SPLogger Logger) {
try
{
_connectionString = getSQLFromSecureStore();
//_logger.WriteLog(SPLogger.Category.High, "LoggingTool", String.Format("Connection string is {0}",_connectionString));
}
catch (Exception ex)
{
Console.WriteLine(ex.Message.ToString());
throw new Exception("Cant be established");
}
}