C# WebClient return an error (401) Unauthorized. Easy fix
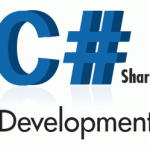
My code in C# console application used to download an image from web service and store it in SharePoint library. But some why the code always returned an error "(401) Unauthorized". The requested URL has been successfully opened in browser on the same machine.
My code was:
string imageFromWebService = "https://server/images/?downloadId=123123"; using (WebClient client = new WebClient()) { imageBytes = client.DownloadData(imageFromWebService); }
Remote server was with Linux OS. And as it turned out, it's the reason.
HttpWebRequest uses the package, it asks for NTLMSSP_NEGOTIATE_SEAL and NTLM_NEGOTIATE_SIGN capabilities. This requres encryption. Since 128bit encryption is now required by the OS, this means that the server also has to support 128bit. If the server doesnt, then the authentication will fail. More information about it you can read here: http://ferozedaud.blogspot.ru/2009/10/ntlm-auth-fails-with.html
You don’t have to change encryption settings to solve the error with authorization. You should just set credential parameter in WebClient:
string imageFromWebService = "https://server/images/?downloadId=123123"; using (WebClient client = new WebClient()) { client.UseDefaultCredentials = true; imageBytes = client.DownloadData(imageFromWebService); }
THANK YOU. 4 days of searching and trying different things.
One blooming line.
Comment by Paul Rivers — July 26, 2018 @ 2:17 pm