C#. How to convert double value to 3 digit group format string
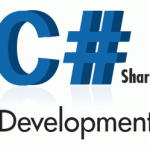
Source: a lot of money values returned from SQL as strings. For example, "1500312.32", "2382938.12", "283727361.10" etc.
The task: display money values in format "1 500 312.32", "2 382 938.12", "283 727 361.10", etc.
Solution:
You can display numeric values in custom formats via namespace "System.Globalization" and its object "CultureInfo".
At first, you need to add "using System.Globalization;" to your code-behind file.
Then you need to create "CultureInfo" object and to override its properties or to create clone and also override them. I advise you to clone CultureInfo object.
And also we need to convert string to double. Don't ask me why it was done so 🙂
The C# code is below
string digits = "1500312.32"; //public CultureInfo(string name, bool useUserOverride); //Create CultureInfo object, choose "en-US" and disallow user override it CultureInfo myCI = new CultureInfo("en-US", false); //Make a clone of en-US CultureInfo object CultureInfo myCIClone = (CultureInfo)myCI.Clone(); //set the number of decimal digits myCIClone.NumberFormat.NumberDecimalDigits = 2; // set the group separator myCIClone.NumberFormat.NumberGroupSeparator = " "; // set size of groups. It can be very custom array like new int[] {2, 3, 5, 1} myCIClone.NumberFormat.NumberGroupSizes = new int[] {3}; //convert double value string to double double idig = 0; try { idig = Convert.ToDouble(digits.Replace(".", ",")); } catch(Exception ex) { Console.WriteLine("Error: " + ex.Message); } string mformat = idig.ToString("N", myCIClone); Console.WriteLine(mformat);