jQuery. Colorize even and odd rows of table
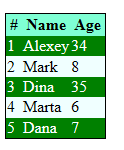
Tables with at least 20 rows are more readable when its rows look like stripes – even row in one color, odd one in another. Before jQuery we used to add class to each row of table, we made "<tr class="even"> …</tr>" and "<tr class="odd"> …</tr>". Now it’s much easier to do using jQuery.
Source data:
Html-code of table:
<table> <tr> <th>#</th> <th>Name</th> <th>Age</th> </tr> <tr> <td>1</td> <td>Alexey</td> <td>34</td> </tr> <tr> <td>2</td> <td>Mark</td> <td>8</td> </tr> <tr> <td>3</td> <td>Dina</td> <td>35</td> </tr> <tr> <td>4</td> <td>Marta</td> <td>6</td> </tr> <tr> <td>5</td> <td>Dana</td> <td>7</td> </tr> </table>
CSS styles for table
<style> table { border: #000 1px solid; border-collapse: collapse; } th { background-color: aquamarine; padding: 2px 4px; } tr { background-color: azure; } tr.striped { background-color: green; color: #FFF; } </style>
To make striped table, we need to add jQuery to a page and add some code:
<script src="js/jquery.js"></script> <script> $(document).ready(function () { $("table tr:nth-child(even)").addClass("striped"); }); </script>
You can make user decide whether he wants to colorize table by himself. In this case you need to add button "Colorize table" and add action "$("table tr:nth-child(even)").addClass("striped");" for it.
<input id="colorizeButton" type="button" value="Colorize table" /> <input id="deColorizeButton" type="button" value="deColorize table" /><br /> <script> $(document).ready(function () { $('#colorizeButton').click(function () { $("table tr:nth-child(even)").addClass("striped"); }); $('#deColorizeButton').click(function () { $("table tr:nth-child(even)").removeClass(); }); }); </script>
# | Name | Age |
---|---|---|
1 | Alexey | 34 |
2 | Mark | 8 |
3 | Dina | 35 |
4 | Marta | 6 |
5 | Dana | 7 |