Open specific tab on page load with JQuery UI
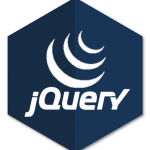
I made a simple HTML page with JQuery UI tabs. But the customer asked to give URLs for each tab. I was a bit surprised with it, because I remember only how to make default tab on page load. You can do it with the syntax like this:
$("#tabs").tabs( { selected: x });
where 'x' is integer index of tab. So, the task was to determine which tab is requested.
I got the collection of tab links (a href='#') and got the values of its links. Also I parsed the URL and got the 'hash' value of URL. Hash has the value after '#'. After that I looked for whether the string of hash is contained in href attributes.
There are two parts of the code - Javascript and HTML. At first, Javascript:
<script>
$(document).ready(function () {
var selectedTab = 0;
var mytabs = $('#tabs ul li a').each(function(x, obj) {
if(obj.hash.indexOf(location.hash) != -1 && location.hash != '') {
selectedTab = x;
}
});
$("#tabs").tabs( { selected: selectedTab });
});
</script>
The second part - HTML code - looks very coomon.
<div id="tabs">
<ul>
<li><a href="#tabs-1">Title of tab 1</a></li>
<li><a href="#tabs-2">Title of tab 2</a></li>
<li><a href="#tabs-3">Title of tab 3</a></li>
</ul>
<div id="tabs-1">
Text for tab-1
</div>
<div id="tabs-2">
Text for tab-2
</div>
<div id="tabs-3">
Text for tab-3
</div>
</div>