Calculate the difference between 2 times in string format in TypeScript
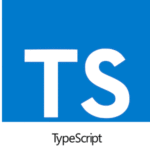
Below you can find a small snippet to calculate the difference between 2 times in string format in TypeScript. If you use JavaScript, remove the types.
For example, you have 2 strings '14:00' and '15:30'. If you use getTimeDiffFromStrings ('14:00', '15:30'), you will achieve '1.5'
/** Function returns the time difference in hours between 2 times in format HH:MM, returns smth like 1.5 */
export const getTimeDiffFromStrings = (startTime:string, endTime:string):string => {
try {
const date1 = new Date(`1970-01-01T${startTime}:00`);
const date2 = new Date(`1970-01-01T${endTime}:00`);
const diffInMs = Math.abs(date2.getTime() - date1.getTime()) / 3600000 ;
return `${diffInMs.toFixed(1)}`
}
catch {
return ''
}
}
getTimeDiffFromStrings('14:00', '15:30') // 1.5