Array in PowerShell – create and read
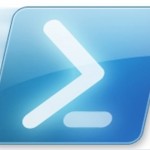
This is an manual how to work with arrays in PowerShell - how to create them, fill and read.
You can initialize array this way:
$ids = New-Object System.Collections.ArrayList
Creating Arrays
To create an Array just separate the elements with commas.
Create an array named $myArray containing elements with a mix of data types:
$myArray = 1,"Hello",3.5,"World"
or using explicit syntax:
$myArray = @(1,"Hello",3.5,"World")
Split the values back into individual variables:
$var1,$var2,$var3,$var4=$
Create an array incremental integer numeric (int) values:
$myArray = 1,2,3,4,5,6,7
or using the range operator (..):
$myArray = (1..7)
or strongly typed:
[int[]] $myArray = 12,64,8,64,12
Create an empty array:
$myArray = @()
Create an array with a single string element:
$myArray = @("Hello World")
Create a Multi-dimensional array (two dimensional):
$myMultiArray = @(
(1,2,3),
(10,20,30)
)
Add values to array
$myArray.Add(2)
$myArray.Add('new item')
Reading array
Return all the elements in an array:
$myArray
Return the first element in an array:
$myArray[0]
Return the fifth element in an array:
$myArray[4]
Return the 5th element through to the 10th element in an array:
$myArray[4..9]
Return the last element in an array:
$myArray[-1]
Return the first element from the first row in a multi-dimensional array:
$myMultiArray[0][0]