5 steps of how to add and use AutoMapper in .Net Core project
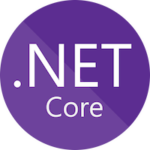
AutoMapper is a simple little library which allows you to make less code for transforming objects of one class to another. It's a must-have library for any complex project. There is a short scenario in 5 steps how to add and use AutoMapper in a new Net Core project with REST API.
1. Manage Nuget Package – AutoMapper. Since version 13 you don't need to use other packages for tge Dependency injection.
2. Register the dependency in Program.cs
builder.Services.AddAutoMapper(typeof(Program).Assembly);
In my previous projects I used
builder.Services.AddAutoMapper(AppDomain.CurrentDomain.GetAssemblies());
3. Create classes sufficiency classes and DTO models. In my example I will use Blog and BlogListItem
public class Blog : BaseClass
{
public string Title { get; set; }
public string Preview { get; set; }
public string TheText { get; set; }
public string ImageUrl { get; set; }
public int? CategoryId { get; set; }
public Category? Category { get; set; }
public Boolean IsDeleted { get; set; }
}
public class BlogListItem : BaseClass
{
public string Title { get; set; }
public string Preview { get; set; }
public string ImageUrl { get; set; }
public string? CategoryName { get; set; }
public int? CategoryId { get; set;}
public Boolean IsDeleted { get; set; }
}
4. Create class with AutoMapper configurations
I created this one for the demo. It must be is inherited from ‘Profile’ class
using AutoMapper;
using AbbContentEditor.Models;
namespace AbbContentEditor
{
public class MapperProfiles : Profile
{
public MapperProfiles()
{
CreateMap<Blog, BlogListItem>();
}
}
}
5. In the controller add Imapper mapper to the constructor and fill the corresponding private class variable.
The example of the usage of the Automapper
var resultDto = _mapper.Map<List<Blog>, IEnumerable<BlogListItem>>(result);
Variable resultDto will be of the type IEnumerable<BlogListItem>.