C# method to create a UNC directory recursively and return the path
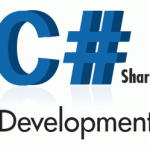
I will write down the useful method. I used it several times and it works good. It's C# method to create a UNC directory recursively and return the path.
It's placed inside a class which I called 'DirectoryCreator'. As a class parameter it receives a path 'workDir'. UNC path in C# must be written just like in Windows - \\AlekseiPC\folder1\.
Method contains a simple RegEx which remove double slash in the end of line.
public class DirectoryCreator
{
private string _workdir { get; set; }
public DirectoryCreator(string workDir) {
_workdir = workDir.ToLower();
}
public string CreateDirectory(string[] folderArray)
{
if (folderArray == null || folderArray.Length == 0) return "Error! empty params";
string dir = _workdir;
foreach (string folderName in folderArray)
{
dir = Regex.Replace(dir, "\\\\$", "", RegexOptions.Compiled);
dir = $"{dir}\\{folderName}\\";
if (!Directory.Exists(dir)) Directory.CreateDirectory(dir);
}
return dir;
}
}
You can call the method like this:
var mainDir = @"\\AlekseiPC\folder1\";
string[] strs = new string[3] { "12", "23", "34" };
DirectoryCreator dc = new DirectoryCreator(mainDir);
string s = dc.CreateDirectory(strs);
Console.WriteLine(s);