Get Groups for AD User with PowerShell or C#
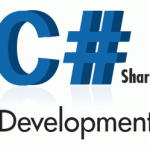
In apps with different Roles and permissions you may need to get information about AD Groups. Here are some snippets which can help you with this part of the task.
Get info about current user in PowerShell
get-aduser -Identity $env:USERNAME
Get AD Groups for current user in PowerShell
Get-ADPrincipalGroupMembership $env:USERNAME | select name
Get the wide information list of Users of the AD Group
If you want to get not only user full name, you can use this command:
Get-ADGroupMember -Identity 'g_zentrale_admin' | Get-ADUser -Properties DisplayName,EmailAddress | Select Name,DisplayName,EmailAddress,SAMAccountName
It gets more data with the sequence of the requests.
Get AD Groups for a user in .Net (C#)
using System.DirectoryServices.AccountManagement;
// ...
using (PrincipalContext pc = new PrincipalContext(ContextType.Domain))
{
UserPrincipal? domainuser = UserPrincipal.FindByIdentity(pc, IdentityType.SamAccountName, userLogin);
if (domainuser == null)
{
Console.WriteLine("Error. No user found");
}
if (domainuser != null)
{
List<string> groups = domainuser.GetGroups().Select(x => x.Name).ToList();
string userDomainGroups = String.Join(", ", groups.ToArray());
Console.WriteLine(userDomainGroups);
}
}
If you use the C# code for SharePoint solution, don't forget to execute it with RunWithElevatedPrivileges()
SPSecurity.RunWithElevatedPrivileges(delegate ()
{
...
});