Get list items from SharePoint 2010 using Client Object model
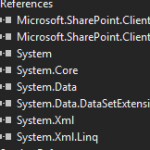
Simple console application, which get data from SharePoint list and displays it. It's very good solution if SharePoint is used as a storage of rates, catalogs, libraries etc.
To use SharePoint Client model you should add references to Microsoft.SharePoint.Client.dll and Microsoft.SharePoint.Client.Runtime.dll in your solution.
Don't forget to add Microsoft.SharePoint.Client.dll and Microsoft.SharePoint.Client.Runtime.dll to GAC (c:\Windows\Assembly) before using application on your PC!
using System; using System.Collections.Generic; using System.Linq; using System.Text; using Microsoft.SharePoint.Client; using SP = Microsoft.SharePoint.Client; namespace msspclient { class Program { static void Main(string[] args) { string siteUrl = "http://spd/site/info/"; ClientContext clientContext = new ClientContext(siteUrl); SP.List oList = clientContext.Web.Lists.GetByTitle("MyList"); CamlQuery camlQuery = new CamlQuery(); camlQuery.ViewXml = @" <View> <RowLimit>100</RowLimit> <Query> <Where> <Eq> <FieldRef Name='CODE' /> <Value Type='Text'>param</Value> </Eq> </Where> </Query> </View> "; ListItemCollection collListItem = oList.GetItems(camlQuery); clientContext.Load(collListItem); clientContext.ExecuteQuery(); foreach (ListItem oListItem in collListItem) { Console.WriteLine("ID : {0} , Title: {1}, BCODE {2} BRANCHNAME {3}", oListItem.Id, oListItem["Title"], oListItem["BCODE"], oListItem["BRANCHNAME"] ); } } } }