How to add test data for the development and tests in EF Core
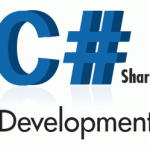
There’s a nice online tool for rendering the test data. It’s at the website https://www.mockaroo.com/. I often use it to render fake SQL data. But now I am working on a Net application with EF Core. And obviously, I need test data for webservices and interfaces. Below is the way to quickly insert the test data in the Net solution.
The idea is to render the test data online, save it as JSON and use it in DbContext class. To add the data in the OnModelCreating you can use the code like this. Obviously, you just need to read the JSON into List<T> and after that iterate the list and add the data to the DB.
string json = File.ReadAllText(@"C:\\Users\\MyAccount\\Downloads\\MOCK_DATA.json");
var locList = JsonConvert.DeserializeObject<List<Location>>(json);
foreach (Location loc in locList)
{
modelBuilder.Entity<Location>().HasData(new Location
{
UserId = loc.UserId,
Capacity = loc.Capacity,
Name = loc.Name,
LocationCategory = loc.LocationCategory,
Id = Guid.NewGuid(),
Address = loc.Address,
District = loc.District,
ResidentGroups = loc.ResidentGroups
});;
}