Regular Expressions in C#. Get text before specified character
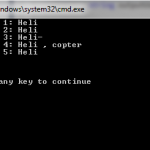
Regular expressions is one of the best ways to work with text. One popular task for Regexp is to get a part of word or expression.Let’s try to get first part from word "Heli-copter", the part that before character "-".
string text = "Heli-copter";
1. Solution with Regex Match everything till the first "-"
Match result = Regex.Match(text, @"^.*?(?=-)");
Comments for solution:
^ match from the start of the string
.*? match any character (.), zero or more times (*) but as less as possible (?)
(?=-) till the next character is a "-" (this is a positive look ahead)
2. Regex Match anything that is not a "-" from the start of the string
Match result2 = Regex.Match(text, @"^[^-]*");
Comments for solution:
[^-]* match any character that is not a "-" zero or more times
3. Regex Match anything that is not a "-" from the start of the string till a "-"
Match result3 = Regex.Match(text, @"^([^-]*)-");
Comments for solution:
This regex will only match if there is "-" in the string, but the result is then found in capture group 1.
4. Convert string to string array using Split and spacer character "-"
string[] result4 = text.Split('-');
Comments for solution:
Result is a Array of strings, the part before the first "-" is the first item in the Array, result4[0]
5. Substring till the first "-"
string result5 = text.Substring(0, text.IndexOf("-"));
Comments for solution:
Get the substring from text from the start till the first occurrence of "-" (text.IndexOf("-")). This works only if there is a "-" in a string, otherwise you get the exception because IndexOf("-") when no "-" return "-1".
Full code here:
string text = "Heli-copter"; Match result1 = Regex.Match(text, @"^.*?(?=-)"); Match result2 = Regex.Match(text, @"^[^-]*"); Match result3 = Regex.Match(text, @"^([^-]*)-"); string[] result4 = text.Split('-'); string result5 = text.Substring(0, text.IndexOf("-")); Console.WriteLine("Result 1: " + result1.ToString()); Console.WriteLine("Result 2: " + result2.ToString()); Console.WriteLine("Result 3: " + result3.ToString()); Console.WriteLine("Result 4: " + string.Join(" , ", result4)); Console.WriteLine("Result 5: " + result5);