Simple implementation of 'env.IsDevelopment' for a console Net Core application
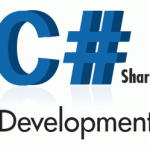
If you work with Net Core WebApi project, it already contains the packages for DI, Environment variables. But if you make a console application from the template, it doesn’t contain the packages for environment variables. And if you want to use different configs for Debug and Release, there are 2 ways:
- remember to write correct ‘appsettings’ file.
- Use environment variables to automize the process.
I prefer to follow the second step. Further is how to do it.
Add the variable ‘ASPNETCORE_ENVIRONMENT’ and set value ‘Development’ in the properties of the project.
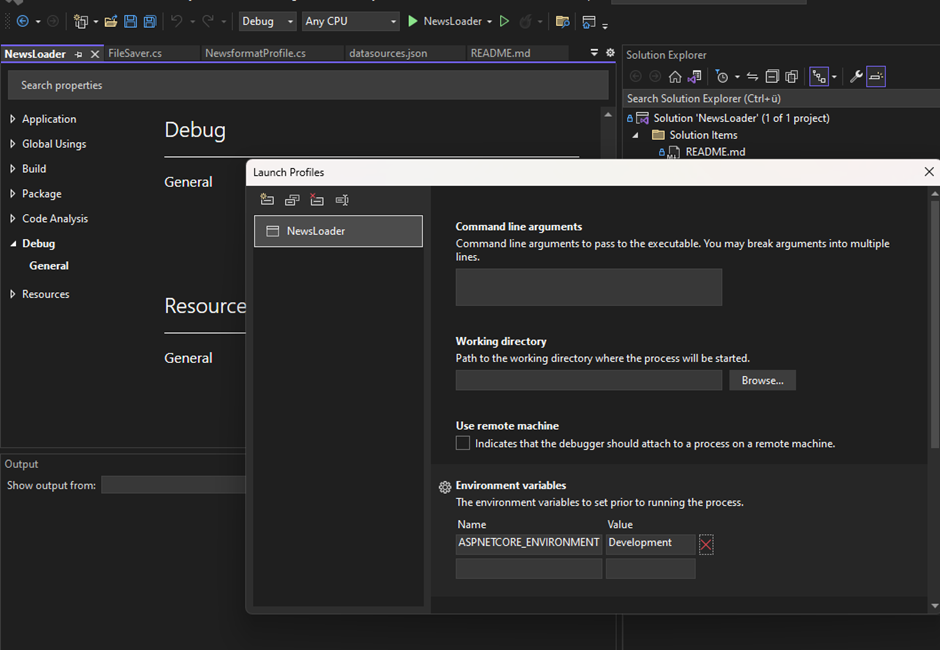
Add code to Program.cs:
var environment = Environment.GetEnvironmentVariable("ASPNETCORE_ENVIRONMENT");
Boolean isDevelopment = environment == "Development";
Console.WriteLine($"isDevelopment: {isDevelopment}");
string configFile = (isDevelopment) ? "appsettings.dev.json" : "appsettings.json";
var configuration = new ConfigurationBuilder()
.SetBasePath(workDir)
.AddJsonFile(configFile, optional: true, reloadOnChange: true);
var config = configuration.Build();
Now if you execute the application with Debug mode, 'appsettings.dev.json' will be used, otherwise 'appsettings.json'
Have a nice coding 🙂