Java. Reading text files
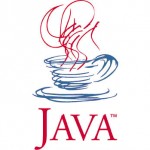
When you created a new project (Java. Create new project in Eclipse), you want to know how to work with text files. Open SampleText.java and add after "package SampleText" two strings.
import java.util.*; import java.io.*;
They will allow us to work with files. Let’s determine the quantity of strings in text file.
String path = "res\\myFile.txt"; // Path to file int i=0; int nums = 0; // Quantity of strings in a file String line = null; System.out.print("Path to file: " + path); try { FileReader fr1 = new FileReader(path); BufferedReader br1 = new BufferedReader(fr1); StringBuilder sb = new StringBuilder(); while ((line = br1.readLine()) != null) { sb.append(line + "\r\n"); i++; } nums = i; System.out.print(nums); System.out.print("\r\n"+sb); } catch (Exception ex) { System.out.print(ex.getMessage()); return; }
This code prints the quantity of lines in file (var nums) and the content of file (var sb). If file is not found, we’ll see the error message.
If we want to read each line of file to String array, we add the code like this:
i = 0; String[] fileStrings = new String[nums]; //declare String array try { FileReader fr1 = new FileReader(path); BufferedReader br1 = new BufferedReader(fr1); while((line = br1.readLine()) != null) { fileStrings[i] = line; i++; } } catch(Exception ex) { System.out.print(ex.getMessage()); return; } for(i=0; i< nums; i++) { System.out.print("\r\nThe string " + String.valueOf(i) + " is " + fileStrings[i] ); }
We declared the string array and set value of it’s element to string values.
If we change try to call the "nums+1" element, we’ll get the error message like this:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 6
at SampleText.SampleText.main(SampleText.java:50)
Using array is a classic method. But quite for a long time instead of arrays it’s better to use collections List<String>. You won't have to think about their sizes.
// TODO Auto-generated method stub String path = "res\\myFile.txt"; // Path to file int i=0; int nums = 0; // Quantity of strings in a file String line = null; System.out.print("Path to file: " + path); List<String> myFileStrings = new ArrayList<String>(); //declare List<string> try { FileReader fr1 = new FileReader(path); BufferedReader br1 = new BufferedReader(fr1); StringBuilder sb = new StringBuilder(); while ((line = br1.readLine()) != null) { myFileStrings.add(line); i++; } nums = i; System.out.print(nums); System.out.print("\r\n"); for (String str : myFileStrings) { System.out.print(str + "\r\n"); } } catch (Exception ex) { System.out.print(ex.getMessage()); return; }