Feed uniqueidentifier field with Random GUID value in SQL
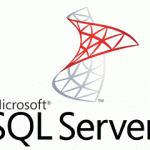
There's a data type 'uniqueidentifier' in SQL. If you need to feed this field with random value, you can use NEWID(). The 'uniqueidentifier' value in SQL looks like this: '234779E5-996F-45AC-90C3-21631AF4A08D'.
If you need to insert records to a table, you can use NEWID() in the statement. For example,
insert into Buildings (id, Nummer, Name, Capacity, Category)
VALUES (NEWID(), '111', 'My private house', 50, 'Internal')
If you need several records, you can write values with comma:
insert into Buildings (id, Nummer, Name, Capacity, Category)
VALUES (NEWID(), '111', 'My private house', 50, 'Internal')
You can also render values with a small tool in any programming language. I use C#:
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 100; i++)
{
string uid = Guid.NewGuid().ToString();
string insertStatement = $"INSERT INTO locations (id, Nummer, Name, Capacity, Category) " +
$"VALUES ('{uid}', '{i}', 'WWW {i}', {i}, 'Category {i}');";
sb.AppendLine(insertStatement);
}
Console.WriteLine(sb.ToString());