Read CSV file to List<T> using CSVHelper
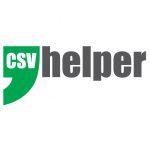
CsvHelper is a very good library for reading and writing CSV files. It’s really easy for coding and fast for work. You can install it via Nuget in Visual studio or download from the project page in GitHub https://github.com/JoshClose/CsvHelper.
To install CsvHelper, run the following command in the Package Manager Console:
PM> Install-Package CsvHelper
Or you can install CsvHelper via Nuget:
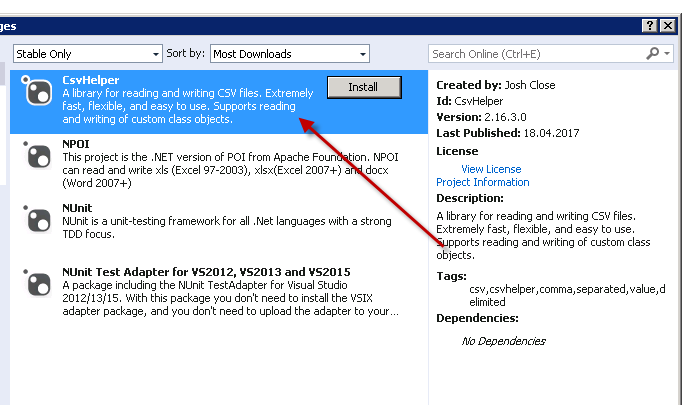
Add CsvHelper to project
Below I pull down a snippet of reading CSV file to generic collection List<StrItem>. Full documentation is published here: http://joshclose.github.io/CsvHelper/
public class StrItem { public string filepath { get; set; } public string theme { get; set; } public string filename { get; set; } } public class CSVRepository { public sealed class StrItemClassMap : CsvClassMap<StrItem> { public StrItemClassMap() { Map(m => m.filepath).TypeConverterOption(CultureInfo.CurrentCulture); Map(m => m.theme).TypeConverterOption(CultureInfo.InvariantCulture); Map(m => m.filename).TypeConverterOption(CultureInfo.InvariantCulture); } } public List<StrItem> Repository() { List<StrItem> records = new List<StrItem>(); string sourceFile = "D:\\Data\\csvfile.csv"; using (TextReader fileReader = File.OpenText(sourceFile)) { var csv = new CsvReader(fileReader); csv.Configuration.HasHeaderRecord = false; csv.Configuration.Delimiter = ";"; csv.Configuration.Encoding = Encoding.UTF8; csv.Configuration.TrimFields = true; csv.Configuration.RegisterClassMap(new StrItemClassMap()); records = csv.GetRecords<StrItem>().ToList<StrItem>(); } return records; } }
And different number of columns per row?
https://stackoverflow.com/questions/47153015/csv-file-with-different-number-of-columns-in-rows
I have one column, int type, contains the number the optional columns. Can be 1, 2, or 10 columns..
Comment by preguntoncojonero — March 6, 2018 @ 1:00 pm