Effective join of array of objects with filtering by condition into a string
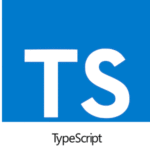
To pass parameters via Get, it was necessary to assemble a string from the selected Checkbox values. In the project, I used Checkbox from Fluent UI, but the approach is similar for another component. Actually, this is not even about React, but about TypeScript.
Task. There is an array of objects. It is necessary to form a line from the elements that were selected by checkboxes. The description of the control and interface is available on the page https://developer.microsoft.com/en-us/fluentui#/controls/web/checkbox
As a result of 2 iterations, the function was reduced to 1 line
const MakeCategoryStr = (newCats:ICheckboxProps[]):string => {
return newCats.filter(x => x.checked).map(x=>x.title).join(',')
}
Detailed example
export interface ICheckboxProps {
title: string,
checked: boolean
}
….
const items:ICheckboxProps[] = [
{checked: true, title: 'Films'},
{checked: false, title: 'Music'},
{checked: true, title: 'Theater'},
{checked: true, title: 'Sport'},
]
const MakeCategoryStr = (newCats:ICheckboxProps[]):string => {
return newCats.filter(x => x.checked).map(x=>x.title).join(',')
}
console.log(MakeCategoryStr(items))
As a result we can see Films,Theater,Sport