Add or remove CSS class of the element in React application
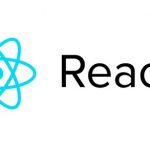
Unlike classic Javascript applications, React works with Virtaual DOM, that’s why it’s not obviously how to add or remove class to the element. I will write about one of the ways how to make it.
For example, you want to make an action with an element when you click it. The default CSS class is ' myCard' and there’s the second class. I suggest you to save class name value in variable which can be updated with useState hook.
In the example below there’s a div with text. When you click it, it is rotated to 180 degrees and after the second click it returns to the previous state.
CSS Code
.myCardWrapper {
width: 400px;
height: 200px;
margin: 0;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
}
.myCard {
border: #153e69 1px solid;
line-height: 50px;
width: 80%;
gap: 20px;
padding: 10px 20px;
transition: 0.5s;
}
.myCard::before {
content: '"';
}
.myCard::after {
content: '"';
}
.myCard180 {
transition: 0.5s;
transform: scaleY(-1)
}
React code
import React, { useState } from 'react';
import './index'
function App() {
const [btnClass, setBtnClass] = useState('myCard')
return (
<>
<div className='myCardWrapper'>
<div className={btnClass} onClick={ () => {
setBtnClass( (btnClass === 'myCard') ? 'myCard myCard180' : 'myCard')
}}>
The card content
</div>
</div>
</>
);
}
export default App;
If you import classes from scss source, you can concatenate values like this:
import styles from './TheFilterForm.module.scss';
// ....
<div className={`${styles.hidden} ${styles.cell}`}>Div content</div>