Get the host of the URL with JavaScript or TypeScript
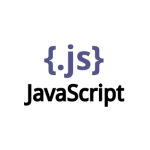
We have a string with an URL. And we want to get the host URL. I don't know about the built-in functions for this and I don't think that you should find external libraries for this. Although, it's a good idea to create your own personal class.
But now I will write down only how to extract the host URL out of the string with full URL.
const _absoluteUrl = "http://mySuperSite.com/sites/Mysites/Files/default.aspx"
First of all, let's split the string with '/' delimiter to have an array of the parts
const hostParts = _absoluteUrl.split('/')
The result is this one
{ hostParts: [ 'http:', '', 'mySuperSite.com', 'sites', 'Mysites', 'Files', 'default.aspx' ] }
As you can see, now we need just to join the 1st and the 3rd elements of the array.
const host = hostParts[0] + '//' + hostParts[2]
That's all folks 🙂 The result is: 'http://mySuperSite.com'
And now let's wrap it in a function
const GetHostFromUrl = (fullUrl) => {
const hostParts = fullUrl.split('/')
if(hostParts.length > 1) return hostParts[0] + '//' + hostParts[2]
return `The string doesn't contain a full URL`
}
const _absoluteUrl = "http://mySuperSite.com/sites/Mysites/Files/default.aspx"
const myhost = GetHostFromUrl(_absoluteUrl)