The implementation of the buttons with Horisontal Scroll action for Material UI DataGrid
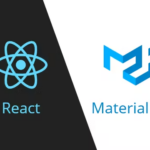
If you use a Pro version of Material UI, then you have a tool, how to switch the cells in the DataGrid table. But if you work with a wide table and you just want to make it more comfortable to work with it, there are some tricks for that. The first thing is to 'freeze' the header, so user can collate the column and headers. All the Excel users like this function. And the second thing is to allow user scroll the page if he has a small resolution and he doesn't have touch screen.
Let's start with the 'freeze' the header function. You override can the DataGrid with parameters and save it in a file. Then you can use it several times in your project.
Create a DataGrid variable with overwritten properties. Add this code to 'StickyDataGrid.ts' file and save.
import styled from "@emotion/styled"
import { DataGrid } from "@mui/x-data-grid"
export const StickyDataGrid = styled(DataGrid)(({theme}) => ({
'& .MuiDataGrid-columnHeaders': {
position: "sticky",
top: 0,
background: 'white',
zIndex: 1000,
},
'& .MuiDataGrid-virtualScroller': {
marginTop: "1 !important",
},
'& .MuiDataGrid-main': {
overflow: "visible",
width: 'auto'
}
}))
You can use the variable in the file with UI. Below I will add the code how to add custom scroll.
You should use 'React.useRef' for that. This hook allows you to access the element directly in the DOM.
In this solution there are 2 buttons which scroll the table by horisontal line. If the scroll is somewhere too far at the bottom, it can be useful.
// ....
const gridRef = React.useRef<HTMLDivElement>(null);
// ....
const handleClick = (position: string) => () => {
if (gridRef.current) {
const t = gridRef.current.getElementsByClassName('MuiDataGrid-virtualScroller')
if (t !== null) {
const currentScrollX = t[0].scrollLeft
if (position.toLowerCase() === 'left') {
t[0].scrollTo({ left: currentScrollX - 200 })
}
if (position.toLowerCase() === 'right') {
t[0].scrollTo({ left: currentScrollX + 200 })
}
}
}
};
<>
<Box sx={{ flexGrow: 1, display: { xs: 'flex', md: 'none' }, position: 'sticky', top: 250, left: 0, zIndex: 1002 }}>
<Button onClick={handleClick('left')}><ArrowCircleLeftIcon /></Button>
<Button onClick={handleClick('right')}><ArrowCircleRightIcon /></Button>
</Box>
<StickyDataGrid
ref={gridRef}
onRowClick={selectGridRow}
showColumnVerticalBorder={true}
scrollbarSize={0} style={{margin: 0}}
showCellVerticalBorder
hideFooter={true}
rows={data}
columns={columns}
sx={{ padding: 0, margin: 0, fontSize: 13,
"& .MuiDataGrid-columnHeaderTitle": {
whiteSpace: "normal",
lineHeight: "normal", fontSize: 12
},
}}
getRowId={(row: any) => row.wnummer}
getRowClassName={getRowClassName}
/>
</>