Update the element from the Array of objects in React + Typescript
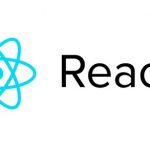
The React snippet of how to update the selected element in the array of objects. The array of objects is connected with useState hook, and on change of Checkbox control the array must be updated.
The method 'setCbStates' is a useState hook for var cbStates:
const [cbStates, setCbStates ] = useState<ICheckboxStates[]>([])
The function which is called onChange of checkbox element to update the array looks like this:
const onSwitchCheckbox = (id: number, checked: boolean) => {
setCbStates ( cbStates.map( elm => {
if(elm.id == id) elm.isChecked = !elm.isChecked;
return elm;
}) );
}
Otherwise you can do it with a new var
const onSwitchCheckbox = (id: number, checked: boolean) => {
const newCbStates:ICheckboxStates[] = cbStates.map( elm => {
if(elm.id == id) elm.isChecked = !elm.isChecked;
return elm;
})
setCbStates ( newCbStates );
}
In this case you can use the new date immediately from this method. Because useState doesn't update the values immediately.