Automating SharePoint List Creation and Indexing with REST API
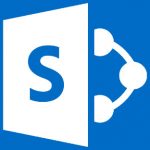
In a recent project, I needed to create a SharePoint list and add an index to it, triggered by the addition of a web part to a page. The goal was to fully automate the process using the SharePoint REST API, eliminating the need for any manual intervention by administrators. Here's how I achieved this.
When a specific web part is added to a SharePoint Online page, a new SharePoint list needs to be created, and an index must be added to this list to enhance query performance. The challenge was to accomplish this using only the SharePoint REST API, ensuring a seamless and efficient solution.
Solution
To achieve this, I implemented a script that leverages the SharePoint REST API to add index to the SharePoint list. There is a lot of scripts how to create a list, but not how to add index.
The most difficult is with headers. Of course, I used pnpjs in my solution. It helped me very much.
import "@pnp/sp/webs";
import "@pnp/sp/lists";
import "@pnp/sp/context-info";
....
createIndex = async (): Promise<boolean> => {
// eslint-disable-next-line no-async-promise-executor
return new Promise( async (resolve, reject) => {
const l2 = await sp.web.getContextInfo()
const requestDigest = l2.FormDigestValue.toLocaleString()
const url = l2.WebFullUrl
const columnName = 'Created By'
fetch(`${url}/_api/web/lists/getbytitle('${this._forecastList}')/fields/getbytitle('${columnName}')`, {
method: 'POST',
body: JSON.stringify( {
"__metadata":{ type: "SP.Field" },
"Indexed":true
}),
headers: {
"IF-MATCH": "*",
"X-HTTP-Method": "MERGE",
'X-RequestDigest': requestDigest,
'Accept': 'application/json;odata=verbose',
'Content-Type': 'application/json;odata=verbose'
}
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
console.log('Index created successfully.', response);
})
.catch(error => {
console.log('Error creating index: ' + error.message);
});
})
}
Probably, this script won't work in your environment as it is, but after minimum of code modifications you can make it work.