Determine with C# if a user exists in the SharePoint group or in the included AD Group
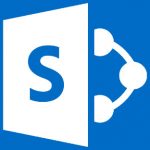
Class to determine if a user is in the SharePoint group or is in the AD Group, which is added to the SharePoint group. So, the admin access to a custom SharePoint module is that everybody, who exists in the admin group, has more data. The admin group is a SharePoint group, which contains SPUsers (from domain) and Domain groups, which contain AD Users. Actual for SharePoint 2016, 2019.
May be there are built-in methods, my colleague told me something about CAML request with memebership, but I could not find one. I used this class in a SharePoint WCF service to restrict data.
public class ADSPUserDetect
{
private readonly string _spweburl;
public ADSPUserDetect(string spwebUrl) {
_spweburl = spwebUrl;
}
public bool GetUserFromGoups(string userName, string spGroupName)
{
bool result = false;
// You can replace using below to "SPSite spsite = SPContext.Current.Site" if you interact with a current Site. Then you don't need a constructor
using (SPSite spsite = new SPSite(_spweburl))
{
using (SPWeb oWebsiteRoot = spsite.RootWeb)
{
var objectsInSPGroup = SPUtility.GetPrincipalsInGroup(oWebsiteRoot, spGroupName, 1000, out bool reachedMaxCount);
bool isUser = objectsInSPGroup.Where(u=>u.LoginName.ToLower().Contains(userName.ToLower())).Count() > 0;
if (isUser) return isUser;
foreach ( var g in objectsInSPGroup.Where(g => g.PrincipalType.ToString().ToLower().Equals("securitygroup")))
{
bool isUserInAd = IsUserInADGroup(userName, g.DisplayName);
if (isUserInAd) return true;
}
}
}
return result;
}
private bool IsUserInADGroup(string userName, string domainGroupName)
{
string[] splittedGroupName = domainGroupName.Split('\\');
string domainName = splittedGroupName[0];
string adGroupName = splittedGroupName[1];
List<string> membersPrincipalNames = new List<string>();
try
{
SPSecurity.RunWithElevatedPrivileges(delegate ()
{
using (var principalContext = new PrincipalContext(ContextType.Domain))
{
using ( var group = GroupPrincipal.FindByIdentity(principalContext, adGroupName))
{
membersPrincipalNames = users.Select(user => user.SamAccountName).Where(x=>x == userName).ToList();
}
}
});
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
return membersPrincipalNames.Count > 0;
}
}
The request to this class (to be honest, to the method) is like this:
ADSPUserDetect userDetect = new ADSPUserDetect(spwebUrl);
bool result = userDetect.GetUserFromGoups("westadmin", "Intern_AdminGroup");
Console.WriteLine(result);
The main method of the class gets the list of users and AD Groups from SharePoint site. If the requested users exists in SharePoint group, the result is true. After that there are requests to found domain groups and the attempt to find the requested user in AD groups. When one is found, it's ok. In all other cases false is returned.