How to set and use environment variables in SPFX solutions (dotenv)
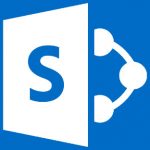
When you work with common modern React application (React > 16) and webpack, it's easy to create .env files and use them in the project. But if you work SPFX solution, which uses GULP, it's a big mess. That's why I decided to describe the sequence of steps how to use different environments in SPFX solutions.
For the example, I write about the WebPart solution.
1. First of all, you need to add dotenv and @types/webpack-env to the solution
npm i dotenv @types/webpack-env
2. Add the code to 'guplfile.js' before 'build.initialize(require('gulp'));'
build.configureWebpack.mergeConfig({
additionalConfiguration: (generatedConfiguration) => {
//use additional logic for env variables
require('./config/env/env')(generatedConfiguration);
return generatedConfiguration;
}
});
3. Create directory 'env' inside the 'config'.
4. Add the file 'env.js' and insert this code:
function setup_env_variables(config) {
console.log(`[env.js]: evnoriment = ${process.env.NODE_MODE}`);
const env_file = `./config/env/.env.${process.env.NODE_MODE}`;
console.log(`[env.js]: injecting data from '${env_file}'`);
const settings = require('dotenv').config({
path: env_file,
});
for (var i = 0; i < config.plugins.length; i++) {
const plug = config.plugins[i];
if (plug.definitions) {
const injected = Object.keys(settings.parsed).reduce((obj, key) => {
obj[`process.env.${key}`] = JSON.stringify(settings.parsed[key]);
return obj;
}, {});
plug.definitions = { ...plug.definitions, ...injected };
}
}
}
module.exports = setup_env_variables;
5. Create env files with variables in 'env' dir, for example, '.env.test'.
Write down the variables and their values, for example
testMode="TEST";
selectedID=12
6. Start the project with setting the environment value
To do it, I add to 'packages.json' (NPM scripts) the line like
"testModeServe": "set NODE_MODE=test&&gulp serve",
and execute it. You can also execute it from command line with the command
set NODE_MODE=test&&gulp serve
7. Use your values
To use values you should use { process.env.selectedID } in your code.
8. Probably you also need to update the 'tsconfig.json' and add 'node' to 'types' property.
"types": [
"webpack-env",
"node"
],