A JS/TS useful snippet to return top N records ordered by time
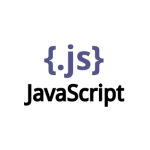
A JS/TS useful snippet to return only several last records with sorting by time. The data is stored in localStorage. I can't modify the types for external component, so that's why all the data has string types. and I use 'moment' to convert the strings to the date format.
maxStored is a constant, it sets the quantity of the elements to store.
const maxStored =5
const limitedMessagess: MessageModel[] = messages.sort( (a, b) => {
return (moment(a.sentTime).toDate() >= (moment(b.sentTime).toDate()) ? 1 : 0)
}).slice(-maxStored)
To understandeasier, there are two operations - the sort and the extract of the elements.
sort() sorts an array in place. This method mutates the array and returns a reference to the same array.
Without parameters it tries to sort by default, it doesn't work with objects or not primitive types.
To sort custom types or objects you should pass the compare function
[11,2,22,1].sort((a, b) => a - b)
It used to determine the order of the elements. It is expected to return:
- a negative value if the first argument is less than the second argument,
- zero if they're equal,
- a positive value otherwise.
slice(start, end) returns a copy of a section of an array. For both start and end, a negative index can be used to indicate an offset from the end of the array. For example, -2 refers to the second to last element of the array.