Horizontal scroll of Div on Button Click in React
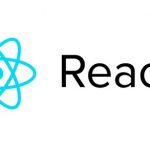
The code of the React component to scroll DIV content by axe X. It can be useful when you have a wide table and a narrow screen. Or a designer had a very cool idea of the interface.
The table looks like this
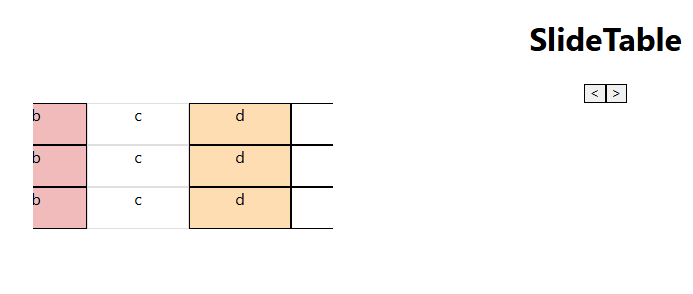
For the implementation I use 'useRef' of React.
export const SlideTable = () => {
const gridRef = React.useRef<HTMLDivElement>(null);
const handleClick = (position: string) => () => {
if (gridRef.current) {
const t = gridRef.current
if (t !== null) {
const currentScrollX = t.scrollLeft
if (position.toLowerCase() === 'left') {
t.scrollTo({ left: currentScrollX - 150 })
}
if (position.toLowerCase() === 'right') {
t.scrollTo({ left: currentScrollX + 150 })
}
}
}
};
return (
<div style={{ paddingLeft: '200px'}}>
<h1>SlideTable</h1>
<button onClick={handleClick('left')}
style={{ border: '#000 1px solid' }}><</button>
<button
onClick={handleClick('right')}
style={{ border: '#000 1px solid' }}>></button>
<div ref={gridRef} style={{ width: '300px', overflowX: 'hidden', position: 'relative', overflowY: 'hidden' }}>
<div className={styles.answerContainer} >
<div className={`${styles.answerblock} ${styles.green}`}>a</div>
<div className={`${styles.answerblock} ${styles.red}`}>b</div>
<div className={`${styles.answerblock} ${styles.passed}`}>c</div>
<div className={`${styles.answerblock} ${styles.orange}`}>d</div>
<div className={`${styles.answerblock} ${styles.pending}`}>e</div>
</div>
<div className={styles.answerContainer} >
<div className={`${styles.answerblock} ${styles.green}`}>a</div>
<div className={`${styles.answerblock} ${styles.red}`}>b</div>
<div className={`${styles.answerblock} ${styles.passed}`}>c</div>
<div className={`${styles.answerblock} ${styles.orange}`}>d</div>
<div className={`${styles.answerblock} ${styles.pending}`}>e</div>
</div>
<div className={styles.answerContainer} >
<div className={`${styles.answerblock} ${styles.green}`}>a</div>
<div className={`${styles.answerblock} ${styles.red}`}>b</div>
<div className={`${styles.answerblock} ${styles.passed}`}>c</div>
<div className={`${styles.answerblock} ${styles.orange}`}>d</div>
<div className={`${styles.answerblock} ${styles.pending}`}>e</div>
</div>
</div>
</div>
);
};